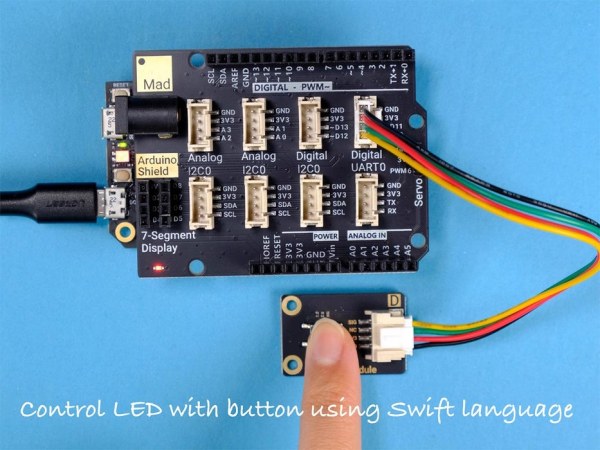
In the two previous projects, the LED turns on and off automatically. Now, you will control the LED manually using a pushbutton.
Step 1: What You Will Need
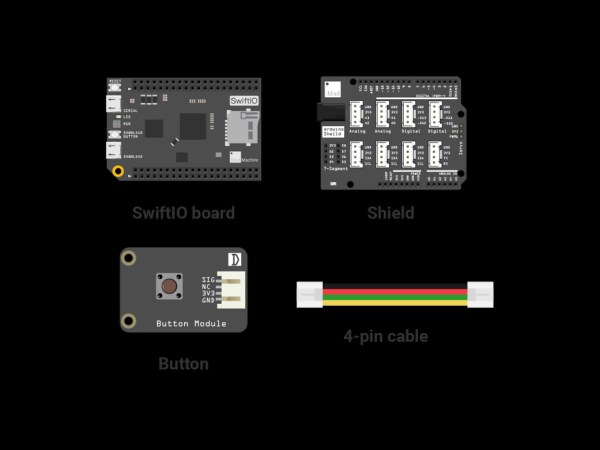
The parts you will need are all included in this Maker kit.
- SwiftIO board
- Shield
- Button module
- 4-pin cable
Step 2: Button
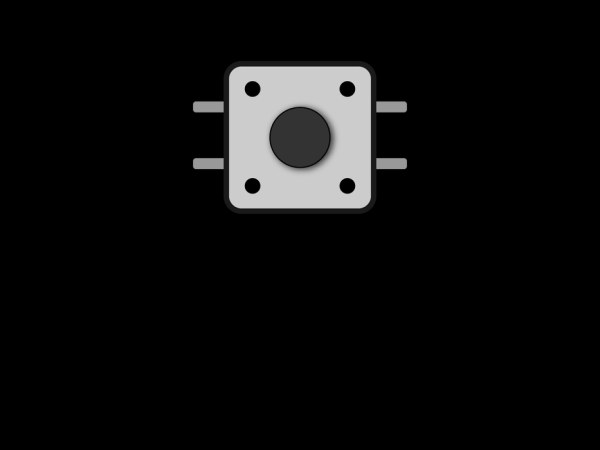
The button, or pushbutton, is always used to control other devices, like the light switch, or the remote control. This button is momentary, so its state will only change as you press it, once you release it, it will go back to its original state.
This kind of button usually has four legs. The two legs on the same side are shorted.
So when you connect a single button, it’s better to connect the two legs on a diagonal line.
While the button module in your kit uses the grove connector, and you could directly build the circuit without worrying about the wrong connection.
Also, there is a known issue with the button: the bounce. Due to mechanical and physical issues, when you slowly press or release the button, there might be several contacts inside the button. And the microcontroller might regard it as several presses. The button module uses the hardware debounce method, so you will not meet with this issue.
Step 3: The Circuit
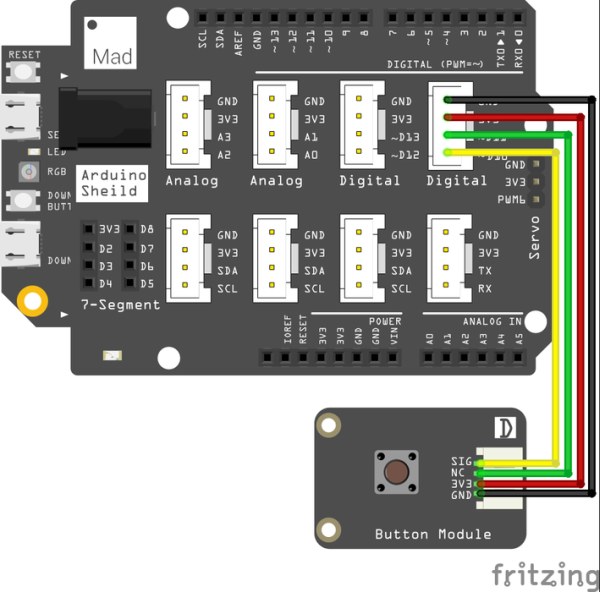
The shield is a modular circuit board that makes it easier to connect the circuit. The pins on the two sides are the same as those on the SwiftIO board. Besides, it has many grove connectors, so you could use a 4-pin cable to connect the pin instead of four jumper wires.
Place the shield on the top of your SwiftIO board. Make sure you connect them in the right direction.
Connect the button module to pin D10 using a 4-pin cable. You could notice each cable has four colors of wires: the black is usually for ground, the red one is for power.
Ok, the circuit is finished. It is really convenient, isn’t it?
Step 4: The Code
// Import the SwiftIO library to use everything in it. import SwiftIO // Import the board library to use the Id of the specific board. import SwiftIOBoard // Initialize the red onboard led. let led = DigitalOut(Id.RED) // Initialize the red onboard led. let button = DigitalIn(Id.D10) while true { // Read the button value. If it is pressed, turn on the led. if button.read() { led.write(false) } else { led.write(true) } sleep(ms: 10) }
Step 5: Code Analysis
import SwiftIO import SwiftIOBoard
First, import the two libraries: SwiftIO and SwiftIOBoard. SwiftIO is used to control the input and output of the SwiftIO board. SwiftIOBoard defines the pin name of the board.
let led = DigitalOut(Id.RED) let button = DigitalIn(Id.D10)
Initialize the red onboard LED and the digital pin (D10) the button connects to.
In the loop, you will use the if-else statement to check the button states. An if-else statement has the following form:
if condition { statement1 } else { statement2 }
The condition has always two results, either true or false. If it is true, statement1 will be executed; if it’s false, statement2 will be executed.
Then let’s look back to the code.
if button.read() { led.write(false) } else { led.write(true) }
The method read() allows you to get the input value. The return value is either true or false. So you could know the states of the button according to the value. If the button is pressed, the value will be true, so the pin output a low voltage to turn on the onboard LED. Once you release the button, the input value is false, the LED will turn off.
Step 6: Run the Project
As you download the code to your board, the red LED is off. If you press the button, the LED turns on. Once you release the button, the LED turns off.
Source: Control an LED With Button Using Swift Language