Summary of TMP36 Temperature Sensor With Arduino in Tinkercad
This article guides building an Arduino-based thermometer using a TMP36 temperature sensor and three LEDs as indicators. The sensor outputs an analog voltage corresponding to temperature, read by Arduino’s ADC on pin A0. The circuit includes three LEDs connected to digital pins 2, 3, and 4 with resistors, lighting up progressively with higher temperature ranges. The article details step-by-step wiring, sensor setup, and code design using block programming and Arduino C code. It explains calibrating thresholds to display temperature levels and encourages experimentation through simulation or physical assembly for interactive thermal readings.
Parts used in the Arduino Thermometer Project:
- Arduino Uno board
- USB cable
- Solderless breadboard
- Three LEDs (any color; red used in example)
- Three 220 Ohm resistors
- TMP36 temperature sensor
- Breadboard wires
For this assignment, your goal is to transform the Arduino into a thermometer! Utilize a temperature sensor to track your skin’s temperature and record the information using three LEDs. The Arduino, being a digital tool, is able to collect information from analog devices such as the TMP36 temperature sensor by utilizing its on-board Analog-to-Digital (ADC) converter, accessible through the well-known analog pins A0-A5.
previous lesson about analog input.
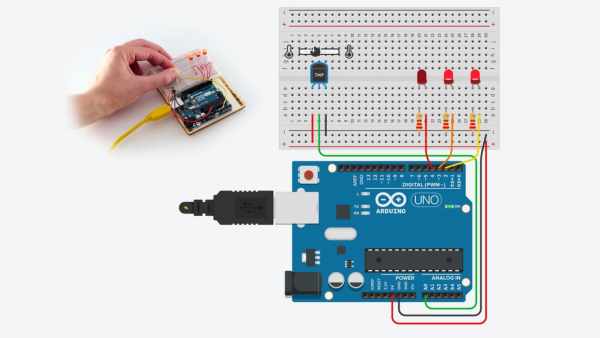
Start by starting the simulation on the circuit and then manipulate the temperature slider on the sensor to observe how it affects the patterns of the LED.
In this tutorial, you will build the simulated circuit on your own while following the provided example. To build the actual circuit, gather an Arduino Uno board, USB cable, solderless breadboard, three LEDs, three identical resistors (any value between 100-1K ohms, with 220 ohms suggested), a TMP36 temperature sensor, and breadboard wires.
You can follow along virtually using Tinkercad Circuits. You can even view this lesson from within Tinkercad (free login required)! Explore the sample circuit and build your own right next to it. Tinkercad Circuits is a free browser-based program that lets you build and simulate circuits. It’s perfect for learning, teaching, and prototyping.
Step 1: Build the LED Circuit
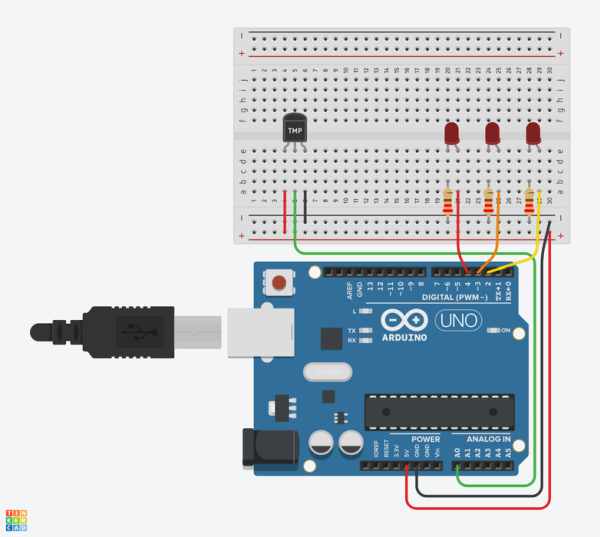
Just as you’ve learned from the introductory lessons, start by wiring up your Arduino and breadboard with power and ground next to the example circuit, then add the the three red LEDs to the breadboard, as shown. These will be the indicator or “bar graph” lights for the project.
Transfer an Arduino Uno and breadboard from the component area to the workspace, next to the existing circuit.
Connect the 5 volt and ground pins from the Arduino to the positive and negative rails on the breadboard with wires. If you like, you can choose to change the wire colors. You have the option to use either the inspector dropdown or the number keys on your keyboard.
Position three LEDs in a line on row E of the breadboard, ensuring a gap of 2 sockets between each LED. Select a LED to access the inspector and change its color.
Link the negative side of each LED (left leg) to the ground line (black) on the breadboard with a 220 Ohm resistor. In Tinkercad Circuits, you can change a resistor’s value by choosing it and using the dropdown menu in the inspector tab.
Connect the longer legs (positive ends) of the LEDs to digital pins 4, 3, and 2 on the Arduino. The point where current enters is the LED anode (+).
The cathode, identified with (-), is where the current begins. This is connected to the ground track.
Step 2: Set up a Thermal Detector.
A temperature sensor generates a voltage signal that changes in response to the sensed temperature. It contains three pins: one for grounding, another for 5 volts connection, and a third that supplies a variable voltage to your Arduino, akin to a potentiometer’s analog signal.
There is a wide variety of temperature sensors available for selection. The TMP36 is beneficial as it produces a voltage output that changes linearly with Celsius temperature.
Locate the temperature sensor within the components drawer of the circuit design tool.
Position the TMP36 temperature sensor on the breadboard with the rounded side facing away from the Arduino, as shown in the example diagram for proper placement.
Position the temperature sensor in row E on the breadboard according to the diagram.
Connect the temperature sensor by linking the left pin to the 5V voltage rail, the middle pin to A0 on the Arduino, and the right pin to the GND rail.
Step 3: Analog Input Observation
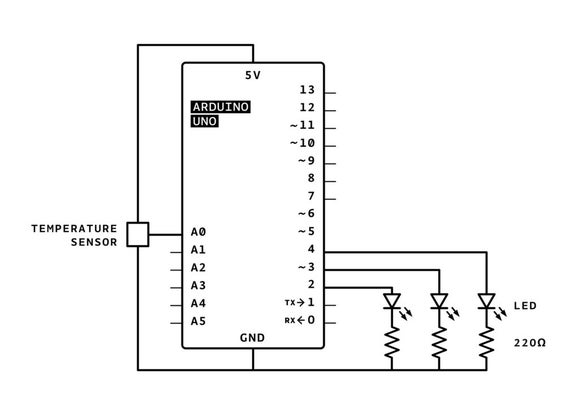
The circuit diagram clearly shows that the temperature sensor is connected to both the 5-volt power source and the 0-volt ground, as well as the analog pin A0. As the temperature rises, so does the voltage on the pin that is linked to A0. It is also noticeable that all three LEDs are connected to separate digital pins.
Even though it is a digital tool, Arduino can still gather information from analog sensors to measure factors like temperature and light. In order to complete this task, you will utilize the Arduino’s built-in Analog-to-Digital Converter (ADC).
Input voltages ranging from 0 to 5V on pins A0 to A5 are transformed into values from 0 to 1023 within the Arduino code. The primary function of the analog pins is to collect information from sensors (and they can also be used as digital outputs 14-19, by the way).
Step 4: Blocks Code
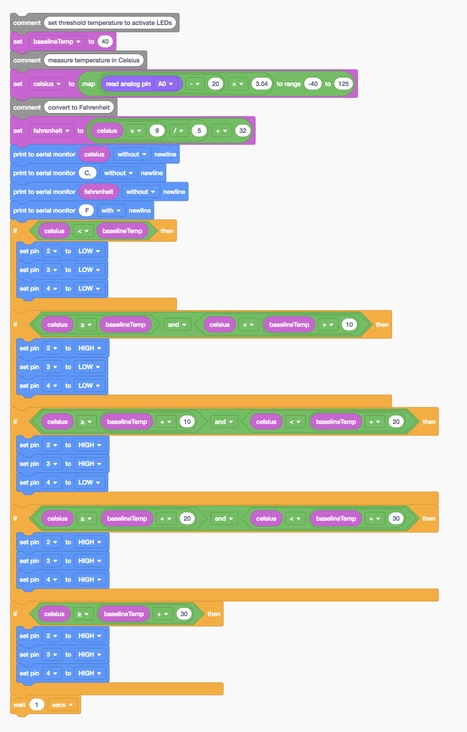
Let’s use the code blocks editor to listen to the state of the sensor, then make decisions about which LEDs to light up based on the sensor’s value.
Click the “Code” button to open the code editor. The grey Notation blocks are comments for making note of what you intend for your code to do, but this text isn’t required or executed as part of the program.
Click on the Variables category in the code editor. Create a new variable called baselineTemp and use a “set” block to set it to 40 (degrees C).
To store the sensor value, create a variable named “celsius”.
Drag out a “set” block and adjust the dropdown to our new variable celsius.
In the Math category, drag out a “map” block, and nest two arithmetic blocks (“1 + 1”) within its first field.
Adjust the range from -40 to 125.
Click on the Input category and drag out an “analog read pin” block, and place it into the first arithmetic field inside the “map” block.
Adjust the arithmetic blocks to “(read analog pin A0 – 20) x 3.04”.
Optionally create a new variable for converting the temperature to Fahrenheit with a set block and some arithmetic blocks to read “set fahrenheit to (celsius x 9)/5 + 32”.
Add some serial monitoring blocks to print out the temperature in one or both C or F.
Click the Control category and drag out an if then block, then navigate to Math and drag a comparator block onto the if block.
In the Variables category, grab the celsius variable and the baselineTemp variable and drag them into the comparator block, adjusting the dropdown so it reads “if celsius < baselineTemp then”.
Add three digital output blocks inside the if statement to set pins 2, 3, and 4 LOW.
Copy and paste this if statement four more times and include arithmetic and logical blocks to have a total of five state detection if statements. In the initial state, LEDs remain unlit due to the temperature being lower than our desired baseline. If the temperature is equal to or higher than baselineTemp but less than baselineTemp plus 10, illuminate the LED on pin 2 exclusively. Illuminate two LEDs when the temperature falls within the range of baselineTemp+10 to baselineTemp+20. And so forth to consider all the required conditions.
Step 5: Arduino Code Explained
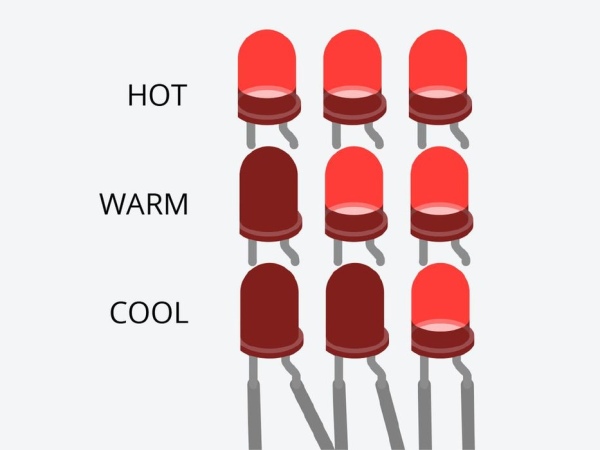
When the code editor is open, you can click the dropdown menu on the left and select “Blocks + Text” to reveal the Arduino code generated by the code blocks. Follow along as we explore the code in more detail.
int baselineTemp = 0; int celsius = 0; int fahrenheit = 0;
Before the setup()
, we create variables to store the target baseline temperature, as well as the sensor value. They’re called int
because they are integers, or any whole number.
void setup() { pinMode(A0, INPUT); Serial.begin(9600); pinMode(2, OUTPUT); pinMode(3, OUTPUT); pinMode(4, OUTPUT); }
Inside the setup, pins are configured using the pinMode()
function. Pin A0 is configured as an input, so we can “listen” to the electrical state of the temperature sensor. Pins 2, 3, and 4 are configured as outputs to control the LEDs.
void loop() { // set threshold temperature to activate LEDs baselineTemp = 40; // measure temperature in Celsius celsius = map(((analogRead(A0) - 20) * 3.04), 0, 1023, -40, 125);
Anything after a set of slashes //
is a comment, just for us humans to read, and is not included in the program when the Arduino runs it. In the main loop, baselineTemp is set to its target 40 degrees C.
// convert to Fahrenheit fahrenheit = ((celsius * 9) / 5 + 32); Serial.print(celsius); Serial.print(" C, "); Serial.print(fahrenheit); Serial.println(" F");
The formula for converting between celsius and Fahrenheit is F = (C * 9) / 5 + 32. Printing to the serial monitor helps you observe the temperature change more granularly than the LED states show alone.
if (celsius < baselineTemp) { digitalWrite(2, LOW); digitalWrite(3, LOW); digitalWrite(4, LOW); } if (celsius >= baselineTemp && celsius < baselineTemp + 10) { digitalWrite(2, HIGH); digitalWrite(3, LOW); digitalWrite(4, LOW); } if (celsius >= baselineTemp + 10 && celsius < baselineTemp + 20) { digitalWrite(2, HIGH); digitalWrite(3, HIGH); digitalWrite(4, LOW); } if (celsius >= baselineTemp + 20 && celsius < baselineTemp + 30) { digitalWrite(2, HIGH); digitalWrite(3, HIGH); digitalWrite(4, HIGH); } if (celsius >= baselineTemp + 30) { digitalWrite(2, HIGH); digitalWrite(3, HIGH); digitalWrite(4, HIGH); } delay(1000); // Wait for 1000 millisecond(s) }
The loop contains six if statements that check various temperature ranges from 40 to 46 degrees C, activating additional LEDs as the temperature increases.
To see a clearer difference in bar graph lights, adjust the baseline temperature variable and/or the range being observed by modifying the if() statements arguments. This process is known as calibration.
Step 6: Use It!
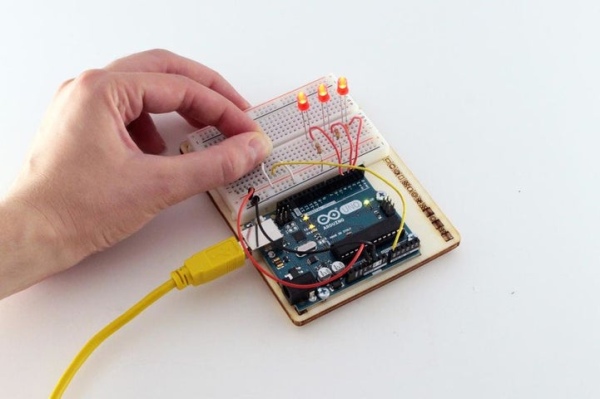
By constructing a physical replica of the circuit, you can experiment with it by using the serial monitor feature in the Arduino software (located in the top right corner of the drawing window) and activating the sensor by touching it with your fingers. If the temperature of the room is extreme or if your fingers are cold, the project may not function correctly.
While utilizing a physical board, keep an eye on the room temperature with the serial monitor and adjust the baselineTemp to align with that measurement.
Change the range of your temperature boundaries to be narrower, such as 2, 4, 6 instead of 10, 20, 30.
Re-upload your code and attempt to grip the sensor using your fingers. Witness the LEDs illuminating in succession as the temperature increases.
Step 7: Next, Try…
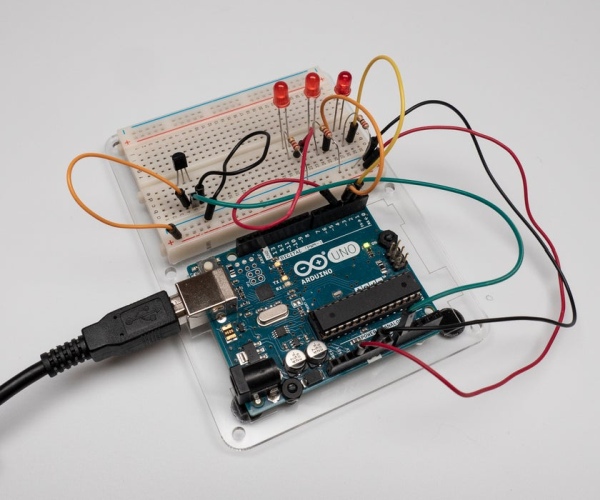
You have used analogRead() and the serial monitor to track changes inside your Arduino and create a simple temperature display with LEDs. You will use this technique with other types of sensors in future projects! One way to expand this project is to create a way for two people to compare finger temperature. Would you need two sensors, or could they take turns? How would you build code to function as well as communicate the way to use it?
You can also learn more electronics skills with the free Instructables classes on Arduino, Basic Electronics, LEDs & Lighting, 3D Printing, and more.
Source: TMP36 Temperature Sensor With Arduino in Tinkercad