This instructable is something like a tutorial for new VB users. It shows how to create a parser base VB6 program to interact with Arduino circuit. Basically, interaction is in the form of serial communication via the USB port. This is my practice after learning VB6 for 2 weeks and hopefully this post can help other newbies in VB6 to see additional options of interfacing this program.
Step 1: Arduino
We must make sure that the Arduino should be able to perform the task of lighting up the LEDs connected. I limited the outputs to six pins because i wanted use the PWM function. The schematic shown above is made from Fritzing Software (Autoroute Option Available)
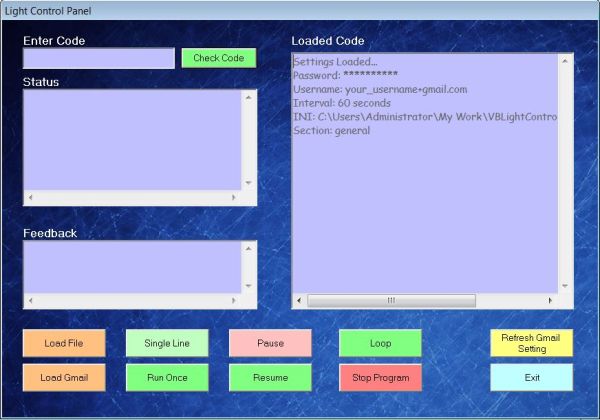
I would like to compliment pwillard as i used some of this parse codes fromhttp://www.arduino.cc/cgi-bin/yabb2/YaBB.pl?num=1293046321 on his “Live For Speed” Racing Simulator. His string manipulation tactic is amazing and just what i needed. I have attached my Arduino Code and feel free to ask if there are any complications. I am also a new learner and just wanted to share what i have achieved so far with Arduino.


Step 2: Visual Basic 6.0 (Part 1)
The second step would be starting to code the VB program. I code some functions as class as this would enable me to create dll. The first class would read text files and store them into an array inside the program. I found the code in “Programming Visual Basic 6.0” manual. This function allows me to load pre-programmed commands into the program to run.
Program Code
Public Function FileToArray(ByVal filename As String) As String
On Error GoTo Error
Dim items() As String, i As Integer
‘ Read the file’s contents, and split it into an array of strings.(Exit here if any error occurs.)
items() = Strings.Split(ReadTextFileContents(filename), vbCrLf)
For i = LBound(items()) To UBound(items())
FileToArray = FileToArray & vbCrLf & items(i)
Next
MsgBox “Commands successfully loaded!”
Exit Function
Error:
MsgBox “Error in FileToArray: ” & Err.Description
End Function
‘read entire context in a file
Public Function ReadTextFileContents(filename As String) As String
Dim fnum As Integer, isOpen As Boolean
On Error GoTo Error_Handler ‘ Get the next free file number.
fnum = FreeFile()
Open filename For Input As #fnum ‘ If execution flow got here, the file has been open without error.
isOpen = True ‘ Read the entire contents in one single operation.
ReadTextFileContents = Input(LOF(fnum), fnum) ‘ Intentionally flow into the error handler to close the file.
Error_Handler: ‘ Raise the error (if any), but first close the file.
If isOpen Then Close #fnum
If Err Then Err.Raise Err.Number, , Err.Description
End Function
After that, i found the program to load inbox messages from Gmail (http://www.j4mie.org/2008/02/15/how-to-make-a-physical-gmail-notifier/ ). I applied this function to enable loading commands from your Gmail inbox to run the Light Controller.
Program Code
Option Explicit
Private m_TheFile As String, m_TheSection As Variant
Private Username As String, Password As String, iTemp() As String
Private pForm As Form, pTimer As Timer, ptxtBox As TextBox, pInet As Inet
Private Declare Function GetPrivateProfileString Lib “kernel32” Alias “GetPrivateProfileStringA” (ByVal lpApplicationName As String, ByVal lpKeyName As Any, ByVal lpDefault As String, ByVal lpReturnedString As String, ByVal nSize As Long, ByVal lpFileName As String) As Long
Private Declare Function WritePrivateProfileString Lib “kernel32” Alias “WritePrivateProfileStringA” (ByVal lpApplicationName As String, ByVal lpKeyName As Any, ByVal lpString As Any, ByVal lpFileName As String) As Long
Public Sub initGmailAccount(TheFile As String, TheSection As Variant, fForm As Variant, fTimer As Variant, ftxtBox As Variant, fInet As Variant)
On Error GoTo ERRR
m_TheFile = TheFile
m_TheSection = TheSection
Set pForm = fForm: Set pTimer = fTimer: Set ptxtBox = ftxtBox: Set pInet = fInet
Log “INI: ” & m_TheFile & vbCrLf & “Section: ” & m_TheSection
pTimer.Enabled = False ‘stop the timer!
pTimer.Interval = SimpleGet(“interval”) * 1000 ‘set the timer!
pTimer.Enabled = True ‘start the timer!
Log “Interval: ” & pTimer.Interval / 1000 & ” seconds”
Username = SimpleGet(“username”)
Log “Username: ” & Username
Password = SimpleGet(“password”)
Log “Password: **********”
Log “Settings Loaded…”
Exit Sub
ERRR:
Log “Error in LoadSettings: ” & Err.Description
Resume Next
End Sub
Public Function CheckMail(ByVal ToTextFile As String) As Boolean
On Error GoTo ERRR ‘error handling. a must.
Dim STRTemp As String ‘in “strtemp” we put the whole web page
Dim mailCount As String, mailTitle As String, mailSummary As String
STRTemp = pInet.OpenURL(“https://” & Username & “:” & Password & “@mail.google.com/gmail/feed/atom”)
STRTemp = UCase(STRTemp)
mailCount = Right(STRTemp, Len(STRTemp) – InStr(1, STRTemp, “FULLCOUNT”) – 9)
mailCount = Left(mailCount, InStr(1, mailCount, “<“) – 1)
mailTitle = Right(STRTemp, Len(STRTemp) – InStr(1, STRTemp, “TITLE>L”) – 5)
mailTitle = Left(mailTitle, InStr(1, mailTitle, “<“) – 1)
If StrComp(mailTitle = “LIGHTCONTROL”, vbTextCompare) = 0 & mailCount = “1” Then
mailSummary = Right(STRTemp, Len(STRTemp) – InStr(1, STRTemp, “SUMMARY”) – 7)
mailSummary = Left(mailSummary, InStr(1, mailSummary, “<“) – 1)
‘load message into public variable
iTemp() = Strings.Split(mailSummary, “;”)
‘save mail data into a textfile
Open ToTextFile For Output As #1
Dim i As Integer
For i = LBound(iTemp()) To UBound(iTemp())
Print #1, iTemp(i)
Next
Close #1
CheckMail = True
Else
Log “Mail not available!!!”
CheckMail = False
End If
Exit Function
ERRR:
Log “Error in CheckMail: ” & Err.Description
Resume Next
End Function
Public Sub Log(Text As String)
On Error GoTo ERRR
ptxtBox.Text = Text & vbCrLf & ptxtBox.Text
Exit Sub
ERRR:
MsgBox “Error while logging: ” & Err.Description
Resume Next
End Sub
Public Function SimpleGet(VarName As String) As String
Static sLocalBuffer As String * 500
Dim l As Integer
l = GetPrivateProfileString(m_TheSection, VarName, vbNullString, sLocalBuffer, 500, m_TheFile)
SimpleGet = Left$(sLocalBuffer, l)
End Function
Public Sub SimplePut(TheItem As Variant, TheVal As Variant)
Call WritePrivateProfileString(m_TheSection, CStr(TheItem), CStr(TheVal), m_TheFile)
‘Flush buffer
Call WritePrivateProfileString(0, 0&, 0&, m_TheFile)
End Sub
For more detail: Arduino & Visual Basic 6 Light Controller