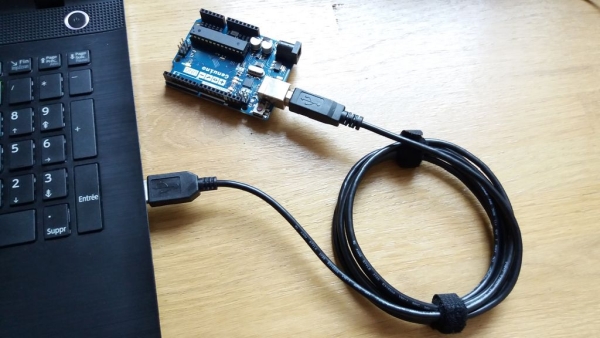
For several projects you might want to acquire some data from an Arduino board. There are several options and the one that I chose here uses the USB connection. I will explain how to store your data in a text file in order to plot it.
I will use Python 3, the Arduino IDE and an Arduino UNO. (I’m working on Linux but I think it should be the same procedure under macOS and Windows.)
Step 1: The Arduino Code
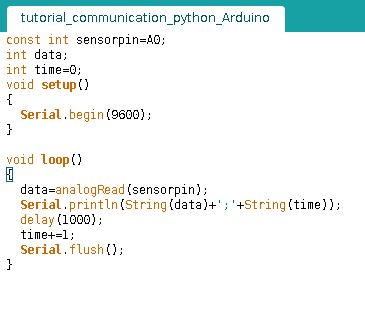
The Arduino will send the data onto the serial connection with the computer as you would the Arduino to send data to the Serial Monitor. So for example you can use the following code. The Arduino takes measures on the A0 analog pin. That’s pretty straight forward !
You can download the Arduino code on My website.
Step 2: Choosing the Proper Port
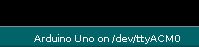
Open the Arduino IDE and try to upload the code you just downloaded. If it worked, copy the port name in the bottom right of the window.
Step 3: The Python Code : Receiving Data
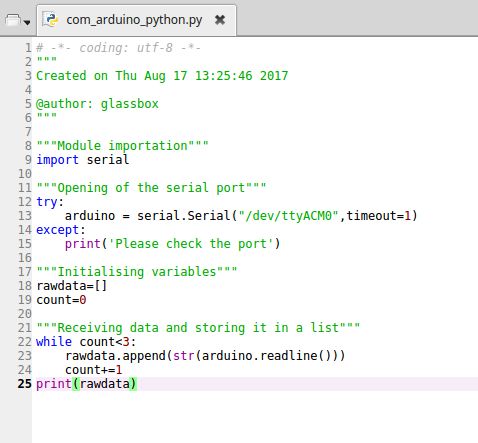
Now we want to receive the data sent by the Arduino with Python (Python3). Therefore we need to install Pyserial.
You can download the Python program on my website
First we import the module serial
Then we try to open a communication; if it’s not possible we print an error.
If you want to change the baudrate it is actually possible like following but make sure it is the same as the one used in the Arduino IDE!
arduino = serial.Serial("/dev/ttyACMO",timeout=1, baudrate=whateverbaudrateyouwant)
Then in the while loop we receive the data. By choosing count<3 we will receive 3 values.
If you just want to print the data without storing it you could use an infinite loop :
while True: print(str(arduino.readline())
But as you can see on the second image the data presents some defects you don’t want to see..
Step 4: Cleaning Up the Data and Storing It in a Textfile
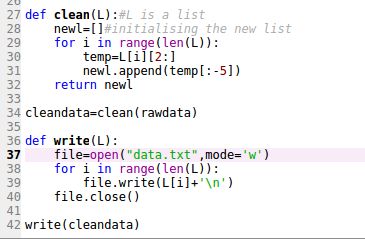
I’ve programmed 2 function allowing me to clean up the rawdata and then writing it in a text file.
I then use numpy.loadtxt with delimiter set on ‘;’ and matplotlib to plot the data.
That’s it ! I hope you found this Instructable interresting and useful.
Download link : As said before all the codes are freely available on my website.
Source: Sending Data From Arduino to Python Via USB