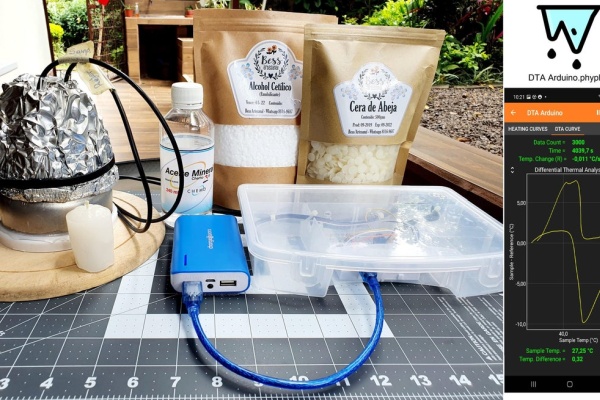
When a solid substance is heated the increase in energy manifests itself in the form of an increase in temperature.
If the heating rate is constant the temperature increase is also constant as long as there is no change in the structure of the substance.
At the time when some kind of transition is initiated in the substance, for example fusion, change of crystal structure or other, the temperature will not increase at the same rate because part of the heating energy will be used in that change of state.
If we compare the temperature of the substance that is experiencing the change of state (Sample) with that experienced by another substance that remains stable (Reference) during heating under identical conditions and subtract a temperature value from another (Sample – Reference) we will be performing what is known as Differential Thermal Analysis (DTA).
The objective of this Instructable is to show the basics of the DTA using Arduino microprocessor, a home heater and a free access program (PhyPhox) that will allow us to obtain on our cell phones curves of Sample Temperature vs Difference (Sample Temperature – Reference temperature) known as thermograms.
Supplies
- Cell Phone (Android or iOS).
- PhyPhox app (Google Play or AppStore).
- 1 Plastic container with multiple compartments.
- 2 Waterproof DS18B20 temperature sensor.
- 2 Breadbord mini modular.
- 1 Arduino Nano V3.0 CH340 with mini usb cable.
- 1 Bluetooth Low Energy BLE CC2541 Bluetooth 4.0 UART.
- 1 1k ohm resistance.
- 1 2k ohm resistance.
- 1 4,7k ohm resistance.
- Male/Male jumpers wire.
- Male/Female jumpers wire.
- 1 Portable battery charger (6000 mAh 3.7V, Out 5V, Output Max. 2.4A).
- 4 Glass test tubes (approximately 1 cm in diameter and 7.5 cm long)
- Wooden base (circular or square of approximately 20 cm in diameter or side).
- 1 Ceiling lampholder (E27).
- 1 Incandescent light bulb of 25 Watts (E27).
- 1 Rotary dimmer switch.
- Approximately 1 m of electrical cable (two cores 0.4 mm thick).
- 1 Two pin electric socket.
- 1 Clothes dryer aluminum duct reducer (10.16 cm to 7.62 cm).
- 2 Disposable circular aluminum plates of approximately 20 cm in diameter.
- 2 Wire insulate screw cap.
- Electrical tape.
- Silicone or Epoxi glue.
- Plastic fasteners.
Step 1: Heating Device
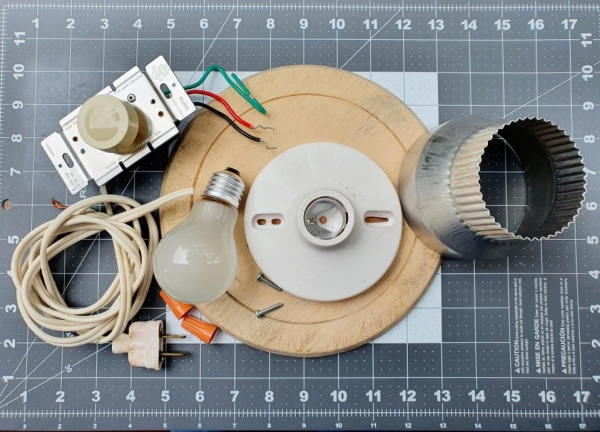
A DTA equipment basically consists of a furnace containing two containers, one for the Sample and one for the Reference and two sensors that measure the temperature of Sample and Reference simultaneously.
The temperature inside the furnace and its heating or cooling rate must be very well regulated so the construction of the furnace in professional equipment is an important factor in the design of a DTA apparatus, however for the illustrative purposes of this Instructable it was chosen to make a rather modest design using homemade materials (see supplies list) as indicated below :
- Fix on a wooden base a ceiling lampholder (E27) provided with two core electrical cable with two pin electric socket.
- Section one of the cores of the electrical cable and connect a rotatory dimmer switch, using wire insulate screw cap or electrical tape to reestablish the cable connection.
- Screw an incandescent light bulb (25 Watts, E27) into the ceiling lampholder.
- With the help of glue, fix on the surface of the ceiling lampholder a clothes dryer aluminum duct reducer (10.16 cm to 7.62 cm) so that the end of smaller diameter is like a chimney around the incandescent light bulb (Caution: Once the bulb is turned on this surface will be hot so there will be a danger of burn).
- Fold the surface of a disposable circular aluminum plates in such a way as to form a concavity that fits on the chimney formed by the clothes dryer aluminum duct reducer (see video in Step 3).
- Using another plate repeat the previous operation forming a concavity that fits on the first and in which two holes near the top of the concavity but separated from each other will be drilled, with a diameter that allows to receive the test tubes (approximately 1 cm in diameter and 7.5 cm long) of Sample and Reference.
- Insert the Sample and Reference test tubes into the drilled holes by pushing until only about 1 cm is left outside. Deform the surface of the aluminum sheet in such a way that they fit on the surface of the test tubes and fix them.
- Hold both aluminum concave covers firm using a plastic fastener.
Step 2: Arduino Connections, Code and Sensor Calibration
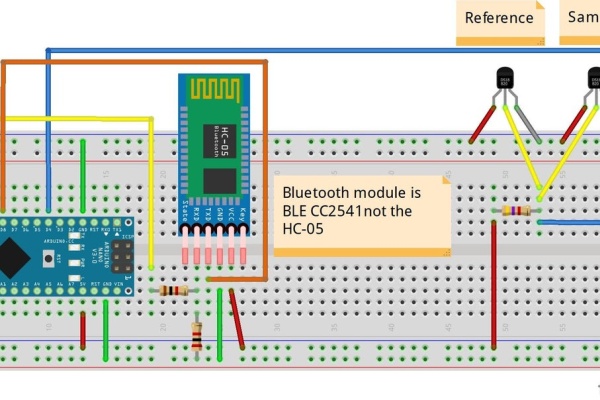
The connections of the to the Arduino controller are shown in a Fritzing scheme that is attached (DTA_Arduino_BLE.fzz). Also attached is the programming code of the Arduino Nano V3.0 CH340 (DTA_Arduino_BLE.ino).
// DTA_Arduino_BLE // IDE 1.8.5 // Adapted and modified by Alberto Villalobos (IDE 1.8.5) // References: // 1. http://hombrosdegigantes.blogspot.com // 2. http://cetroniconline.blogspot.com/2014/07/tutorial-arduino-iv-sensor-de.html?m=1 // 3. https://randomnerdtutorials.com/guide-for-ds18b20-temperature-sensor-with-arduino/ // 4. http://diymakers.es/arduino-bluetooth/ #include <AltSoftSerial.h> #include <OneWire.h> #include <DallasTemperature.h> float CorrTempRef = 0.37; //Correction to the temperature initially measured by the Reference sensor float CorrTempSamp = 0.69; //Correction to the temperature initially measured by the Sample sensor AltSoftSerial BTserial(9, 8); // RX | TX // Data wire is conntec to the Arduino digital pin 4 #define ONE_WIRE_BUS 4 OneWire oneWire(ONE_WIRE_BUS); DallasTemperature sensores(&oneWire); // ***** Indicate the directions of the sensors (each DS18B20 sensor has its own code) ***** DeviceAddress Ref = {0x28,0xFF,0x68,0xDD,0x64,0x15,0x02,0x6F}; // See References 1 and 2 DeviceAddress Samp = {0x28,0xFF,0x2E,0x90,0x64,0x15,0x02,0x67}; // See References 1 and 2 void setup(void) { Serial.begin(9600); BTserial.begin(9600); sensores.begin(); } void loop(void) { sensores.requestTemperatures(); float TempSensorRef = sensores.getTempCByIndex(Ref)+CorrTempRef;//Reference Sensor float TempSensorSamp = sensores.getTempCByIndex(Samp)+CorrTempSamp;//Sample Sensor // ***** Establish the resolution for each sensor ***** //9 bits 0.50 ºC //10 bits 0.25 ºC //11 bits 0.125 ºC //12 bits 0.0625 ºC sensores.setResolution(Ref,12); sensores.setResolution(Samp,12); Serial.print(TempSensorRef);Serial.print(",");Serial.println(TempSensorSamp); BTserial.print(TempSensorRef); BTserial.print("/"); BTserial.print(TempSensorSamp); BTserial.print("/"); delay(500); // Determines sensor data capture rate }<br>
DS18B20 Sensor Calibration:
It should be noted in this code that the float CorrTempRef and CorrTempSamp constant are corrections that should be applied to the initial measurements of the DS18B20 temperature sensors as a calibration and that it must be determined for each individual sensor being used.
float CorrTempRef = 0.37; //Correction to the temperature initially measured by the Reference sensor float CorrTempSamp = 0.69; //Correction to the temperature initially measured by the Sample sensor
This correction is estimated by reading the temperature value that the sensor marks when immersed in an ice bath (ice in the presence of liquid water). The deviation of 0°C, which is the value that the ice bath should have, is the one used as a temperature sensor correction.
In this case the measured correction for the Reference and Sample DS18B20 temperature sensors was 0.37°C and 0.69°C respectively and the data were read directly from the designed PhyPhox app (see Step 3) value then used to define the correction in the Arduino code.
DT18B20 Addresses:
The DS18B20 temperature sensors work with OneWire technology which means that several sensors can be connected to the same digital port since each sensor has a factory code (e.g. 0x28 0x8A 0xB1 0x40 0x04 0x00 0x00 0xC7) that allows the Arduino to recognize the reading that each sensor makes independently.
This is very advantageous because it saves digital ports, especially when the project requires multiple sensors.
In our case, as seen from the Arduino circuit diagram, the two DS18B20 temperature sensors are connected to a single digital port (pin 4) which is why it is necessary to find out the particular code for each one.
To find out the address of each sensor you must assemble a circuit to read this code (DS18B20_Address.fzz), load the code DS18B20.ino and test individually each sensor.
// DS1820_Address // IDE 1.8.5 // Adapted and modified by Alberto Villalobos (IDE 1.8.5) // References: // http://cetroniconline.blogspot.com/2014/07/tutorial-arduino-iv-sensor-de.html?m=1<br> #include <OneWire.h> #include <DallasTemperature.h> #define ONE_WIRE_BUS 4 OneWire oneWire(ONE_WIRE_BUS); DallasTemperature sensores(&oneWire); DeviceAddress Sensor; void setup(void) { Serial.begin(9600); Serial.println("Address Sensor DS18B20:"); sensores.begin(); if (!sensores.getAddress(Sensor, 0)) //If it is not possible to determine the address gives us an error message Serial.println("Unable to find sensor address."); } //Create the show Address function void Mostrar_Direccion(DeviceAddress direccion) { for (uint8_t i = 0; i < 8; i++) { Serial.print("0x"); if (direccion[i] < 16) Serial.print("0"); Serial.print(direccion[i], HEX);//Usamos formato Hexadecimal } } void loop(void) { Serial.print("Address: "); Mostrar_Direccion(Sensor); Serial.println(); delay(5000); }
The code or address obtained must then be separated with commas every four characters. For the two DS18B20 sensors used in this Instructable the directions determined were as follows:
Reference = 0x28,0xFF,0x68,0xDD,0x64,0x15,0x02,0x6F
Sample = 0x28,0xFF,0x2E,0x90,0x64,0x15,0x02,0x67
Step 3: PhyPhox Parameters and Installation
PhyPhox is an application that allows design your own Experiments using cell phone sensors, external sensors (connected via bluetooth) or both, through an online editor (Editor-Test) in which you can define relationships between the captured data, graph them and save the results.
This is the case of this Instructable in which an PhyPhox app (DTA Arduino.phyphox) was designed to read the temperature values measured by the DS18B20 sensors, one in the Reference substance and another in the Sample under analysis.
With the data measured by the sensors, the analysis of the information is performed by the PhyPhox app installed on the cell phone which makes the device designed independent of a desktop or laptop computer.
In this way, the measured data are plotted with respect to time which allows to visualize the behavior of the temperature of both Reference and Sample over time.
The result of the subtraction of the Sample temperature minus the Reference as well as the value of this difference vs the sample temperature is also plotted.
This last graph, called a thermogram, will allow us to visualize the state changes that occur in the sample both when heating it and when letting it cool, which is the purpose of this Instructable.
The specific parameters that make up the experiment designed in this Instructable (DTA Arduino.phyphox) are shown in the video attached to this Step as well as the procedure necessary for loading experiment on the cell phone.
Details on how to design an experiment using online editor for PhyPhox can be found in the Experiment Editor PhyPhox website. While the process of designing the Experiment is quite intuitive I would like to place special emphasis on some points which, in my experience, were crucial to making communication between the Arduino and the cell phone using PhyPhox.
The above points are related to the Input tab of the Editor-Test and have to do with Bluetooth device parameters called “Device Name” and “characteristic“. To find out these parameters Arduino microprocessor connections listed in Step 2 must be made and the armed equipment connected to the portable battery.
Under these conditions the Bluetooth device will start issuing information, including the parameters we want to find out. There are many mobile phone applications that allow us to access this information, in our case one was used for Android called BLE Analyser (free with advertisements) that allowed us to recognize the Name of our device as well as the Custom Characteristic that is the one we specifically need (see explanatory image in this Step).
There is another parameter that we need to know and that is also requested in the Input tab of the Editor-Test, is the so-called “separator” and that relates to the character used by Bluetooth to separate the data that is transmitted. This character is defined in the programming of the Arduino file and in our case is set with the symbol “/” (see explanatory image in this Step).
Pro Tip 1: A simple way to understand how the online editor works is to load Experiments to the Editor-Test from those included in the app.
Pro Tip 2: PhyPhox is designed to use low-energy bluetooth emitters (LBE) so you need to ensure the use of this kind of device.
Source: Differential Thermal Analysis Using Arduino and PhyPhox